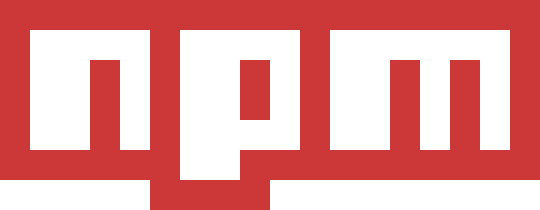
1. Create a Script in package.json
In your package.json
, define a script inside the "scripts"
section. Let’s say you want a script called myScript
:
{
"scripts": {
"myScript": "node script.js"
}
}
This script simply runs a Node.js file, script.js
. Now, we can send arguments to this script.
2. Sending Command-Line Arguments
You can pass arguments to an npm
script by appending --
followed by the arguments. Anything after --
is forwarded to the script:
npm run myScript -- arg1 arg2 arg3
For example:
npm run myScript -- hello world 123
Without --
, the arguments will not be passed to your script!
3. Accessing Arguments in the Script
In Node.js, you can access command-line arguments using process.argv
. The first two entries are the Node.js executable and the script path, so the actual arguments start from index 2
.
// script.js
const args = process.argv.slice(2); // slice(2) to skip node and script path
console.log(args); // ['hello', 'world', '123']
4. Example Usage
- Add the script in
package.json
:
{
"scripts": {
"myScript": "node script.js"
}
}
- Create
script.js
:
// script.js
const args = process.argv.slice(2);
console.log('Arguments received:', args);
- Run it with arguments:
npm run myScript -- foo bar baz
This will output:
Arguments received: [ 'foo', 'bar', 'baz' ]
5. Passing Flags
You can also pass flags and options:
npm run myScript -- --name=John --age=30
In script.js
, you can then access these as:
// script.js
const args = require('minimist')(process.argv.slice(2));
console.log(args); // { _: [], name: 'John', age: 30 }
Here, minimist
is a popular package to parse flags and arguments.
Summary:
- Use
--
to pass arguments tonpm
scripts. - Access the arguments in the script via
process.argv
. - Optionally use a library like
minimist
for easier parsing of flags.
Let me know if you’d like more details!